How to Integrate an External Media Provider with Duda's Media Picker
Duda enables partners to seamlessly integrate their media libraries into the Media Picker experience inside the website editor. This allows users to browse, search, and add third-party media directly into their site projects.
Objective and Outcomes
This guide walks you through the standard REST API implementation required for your service to become a provider in Duda’s ecosystem. We’ll cover:
- Required endpoints and how Duda interacts with them
- Authentication setup
- Integration lifecycle
- Best practices
- Example API responses and request formats
How the Integration Works
Your service exposes a secured REST API, which Duda interacts with to:
- Detect supported features (
/info
) - Load and search your images (
/search/images
) - Fetch image metadata when a user selects an image (
/images/{id}
)
Duda initiates these calls based on user interactions inside the Media Picker. Your image results can be shown above or below other providers like Pexels , or can be configured as the only available choices, offering users a premium curated experience.
Authentication
All requests made by Duda will include a custom header you specify during onboarding. This information must be provided to your AM to be configured in your account by Duda. You provide:
auth_header_name
(e.g.x-api-key
orAuthorization
)auth_header_value
(e.g. a bearer token or static key).
NOTE: This authentication header can be called whatever you want, as long as you provide Duda the key.
GET https://partner.example.com/info
x-api-key: abc123securekey
Duda securely stores this information and includes it in every API call.
Required Endpoints
Note: All endpoints must be served over https.
1. GET /info
GET /info
Returns capabilities and branding for your provider. Currently only the "IMAGE" resource_type and "BY_QUERY" search_images_capability is supported. Below is a sample call and response:
curl -X GET "https://partner.example.com/info" \
-H "x-api-key: abc123securekey"
{
"provider_details": {
"name": "Unsplash Pro",
"logo": "https://cdn.example.com/logo.png",
"link": "https://unsplash.com"
},
"search_capabilities": {
"resource_types": ["IMAGE"],
"search_images_capabilities": ["BY_QUERY"]
}
}
2. GET /search/images
GET /search/images
Returns images based on a query or pagination request. Note the final path segment (images) matches the resource type(s) returned from the info endpoint. Currently only images are supported but other media types may be added in the future, requiring several endpoints. Below are the listed query parameters:
query
(optional - The actual search term typed into the Duda media picker)page
(required, starts at 1)per_page
(required)
Below is a sample call and response:
curl -X GET "https://partner.example.com/search/images?query=dog&page=1&per_page=10" \
-H "x-api-key: abc123securekey"
{
"images": [
{
"id": "img_901",
"name": "img_901.jpg",
"urls": {
"original": "https://cdn.example.com/images/901.jpg",
"preview": "https://cdn.example.com/images/901_preview.jpg",
"small": "https://cdn.example.com/images/901_small.jpg"
}
}
],
"page": 1,
"per_page": 10,
"total_results": 100
}
3. GET /images/{id}
GET /images/{id}
Retrieves metadata and the original
image URL. Below is a sample call and response:
curl -X GET "https://partner.example.com/images/img_901" \
-H "x-api-key: abc123securekey"
{
"id": "img_901",
"name": "img_901.jpg",
"urls": {
"original": "https://cdn.example.com/images/901.jpg",
"preview": "https://cdn.example.com/images/901_preview.jpg",
"small": "https://cdn.example.com/images/901_small.jpg"
}
}
Name must equal the filename with extension
The name field specifies the full filename (including extension) of the image, such as banner.jpg or icon.png. This value determines the image type displayed in the Duda Media Picker and must include the file extension. The name field is required in responses from both the
/search/images
and/images/{id}
endpoints.
For more information, check out the REST API definition in the form of Open API yaml:
- Navigate to https://editor.swagger.io/
- File→Import URL →https://editor-sandbox.duda.co/api/public/partner-rest-resources-provider/api-docs/yaml
- The complete development documentation will be presented on the right
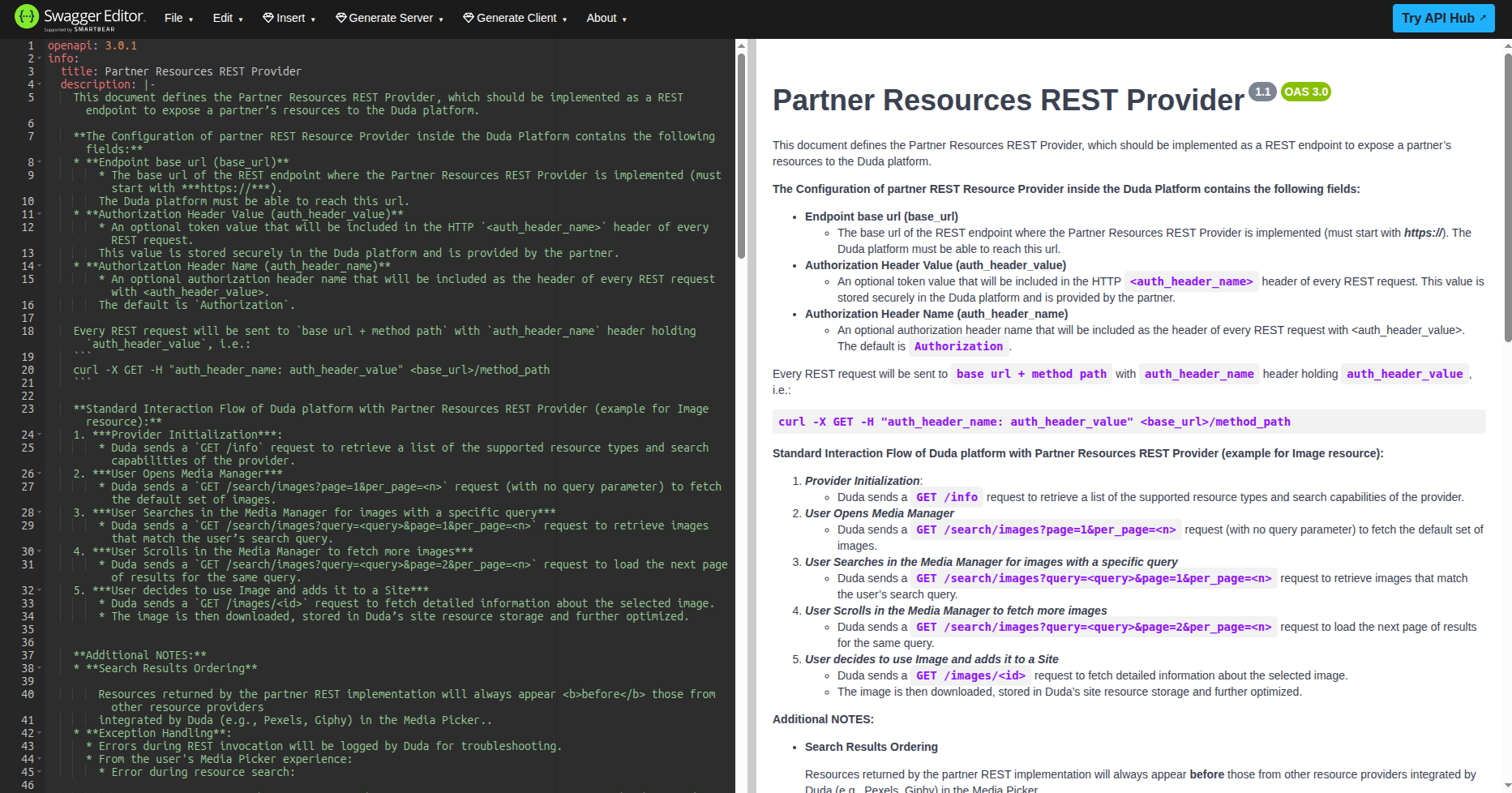
Error Handling
- Search Failures: Partner media results are skipped silently; fallback providers are shown.
- Media Retrieval Failures: The user is shown an error when trying to add the media to their site.
Testing and Onboarding
- Implement the API per the above requirements
- Share your base URL, authentication header name and value with Duda
- Duda manually verifies your implementation
- Once approved, log into your account to test the Media Picker experience
Tips for a Great Integration
- Use CDN-optimized image URLs for fast delivery
- Provide high-resolution previews and thumbnails
- Ensure pagination metadata is accurate
- Respond quickly to queries to avoid timeouts in the Media Picker
Updated 23 days ago